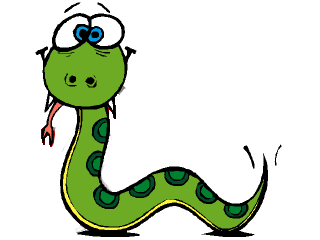
I’ve been recently experimenting with a new string formatting library for C++ and realized that it can be used for converting objects to strings à la Python’s str function. In fact the implementation of such function is almost trivial:
template <typename T>
std::string str(const T &value) {
return fmt::format("{}", value);
}
See this post
to learn more about fmt::format
.
The str
function, unlike sprintf
, can work with any type that has
appropriate std::ostream
inserter operator <<
. For example:
class Date {
int year_, month_, day_;
public:
Date(int year, int month, int day)
: year_(year), month_(month), day_(day) {}
friend std::ostream &operator<<(std::ostream &os, const Date &d) {
os << d.year_ << '-' << d.month_ << '-' << d.day_;
return os;
}
};
auto s = str(Date(2012, 12, 9));
// s == "2012-12-9"
The str
function applies the default formatting for the type, so
if you want to have control over formatting you should use format
instead.
Being based on format
, str
uses IOStreams only for user-defined types,
but not for built-in types which it handles
much more efficiently.
Additional performance improvement in str
can be achieved by getting rid
of the format string and passing arguments to the formatter directly.
This requires minor changes to the library since this functionality is
currently private to the Formatter
class.
Last modified on 2012-12-17